Welcome to this edition on how to build an Android app from scratch. We will learn how to create an Android file to Excel converter.
I will be explaining the step-by-step process I used to create this app. A copy of this app has been published in the Google Play Store. If you want to have a feel of what we will be working on, feel free to check out the app with this link.
Creating an Android app from scratch may be easy or hard, depending on the kind and features of the app.
Below are some of the app screenshots in the Google Play Store.
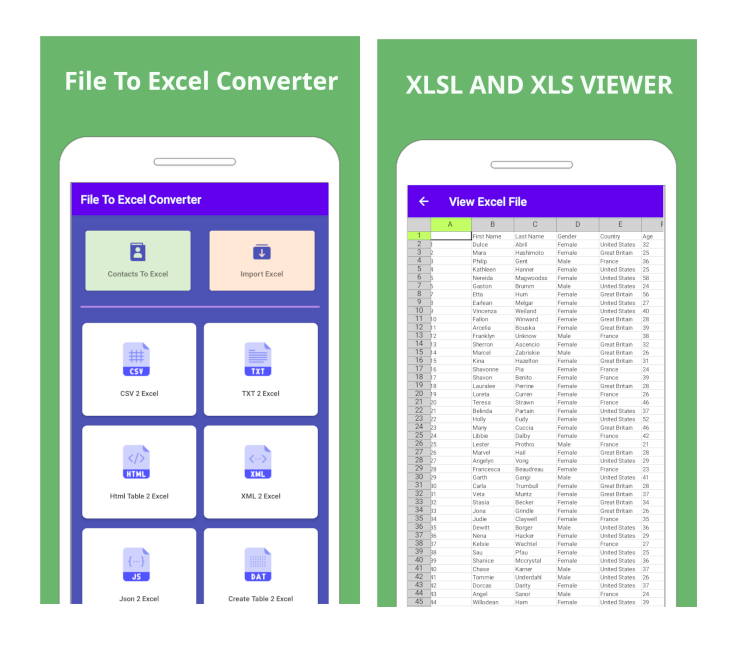
The App Features
Before writing code, we need to understand the app features and what we can do with the app. As the name implies, an Android File to Excel Converter is a simple utility app that converts different file formats to Excel files.
- XLSL and XLS Viewer: Open and view any Excel file directly on your phone.
- Import Excel: Import the Excel file and add new rows and columns to the file.
- Convert Phone Contacts to Spreadsheet: Provides easy ways to select contacts from your contact list and convert it to Excel.
- Convert CVS to Excel: View and convert CVS files in your device to Excel.
- Text File to Excel: Convert the text file to Excel.
- Convert HTML file to Excel: Easy convert HTML tables to Excel Files.
- Convert XML to Spreadsheet: Convert any XML file to Spreadsheet with a button click.
- Convert JSON file to Excel.
- Convert Custom and Template Tables to Excel: You can create a table of your kind and convert it to Excel.
- Convert Spreadsheet to PDF: Easily convert Spreadsheet files to PDF.
- Print Spreadsheet files.
- Export Spreadsheet Files to different file extensions.
Android File Formats To Excel Supported
So far, the Android Excel Converter application only supports the following file formats to Excel files. There are plans in the future to support more file formats.
- Android Contacts to Excel Converter.
- Android CSV to Excel Converter.
- Android TEXT to Excel Converter.
- Android HTML to Excel Converter.
- Android JSON to Excel Converter.
- Android Custom Table to Excel Converter.
Android Excel Convert App UI Design
For this tutorial, we will maintain the same UI design as seen in the Google Play Store. Please kindly download the app if you have not.
Creating A New Project in Android Studio
I will not cover how to create a new Android Project in Android Studio since many tutorials have done that already.
You can choose a name for the following.
- Package Name
- App name
For this tutorial, we will use Java for Android. You can reproduce the code in Kotlin if you want to use Kotlin. This tutorial is for learning purposes.
Once you create a new Android project, we will add an intro page that will load when the app opens.
The intro page is the only page we will create on creating an Android app from scratch introductory tutorial. Subsequent tutorials will cover each of the topics listed above.
Create An Intro Activity Page
- Open your project in Android Studio, create a new Activity page, and name it IntroActivity or your choice name.
- Open the new file, copy and paste the code below.
- The Android Handler class delays code execution for 2 seconds.
- After the delay, it navigates the user to a new page. We will create the new page in the coming tutorial.
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//initInterstitialAd();
new Handler().postDelayed(() -> {
gotoHomeActivity();
}, 2000);
}
private void gotoHomeActivity(){
Intent homeIntent = new Intent(MainActivity.this, HomeActivity.class);
startActivity(homeIntent);
Bungee.slideLeft(MainActivity.this);
finish();
}
}
Create Intro Activity Layout File
- Open the intro activity XML layout file and paste the code below to the file.
- Feel free to redesign the layout to give it your personal touch.
<?xml version="1.0" encoding="utf-8"?>
<androidx.appcompat.widget.LinearLayoutCompat xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
android:background="@color/notice"
tools:context=".MainActivity">
<androidx.appcompat.widget.LinearLayoutCompat
android:id="@+id/image_row"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:layout_marginTop="120dp">
<androidx.appcompat.widget.AppCompatImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/excel"
android:scaleType="centerCrop"/>
</androidx.appcompat.widget.LinearLayoutCompat>
<FrameLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<androidx.appcompat.widget.LinearLayoutCompat
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="12dp"
android:gravity="center"
android:orientation="vertical">
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="18sp"
android:layout_marginTop="12dp"
android:text="Excel Converter and Viewer"
android:textStyle="bold"
android:textAllCaps="true"
android:textColor="@color/purple_500"/>
<androidx.appcompat.widget.AppCompatTextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="12sp"
android:layout_marginTop="12dp"
android:textStyle="italic|bold"
android:textColor="@color/black"
android:text="Convert PDF, Image, CSV, Word and Contact Tables to Excel"/>
</androidx.appcompat.widget.LinearLayoutCompat>
</androidx.appcompat.widget.LinearLayoutCompat>
Res/Values Folder
Go to the res > values folder and update the strings.xml and colors.xml files.
That is all for now. We will create almost ten tutorials in this series. The Android Excel Converter app is the end product of this series. As we go further with each tutorial, we will introduce new concepts and elaborate on each design and code choice.
If you have an example Android app you want us to recreate from scratch and write a comprehensive tutorial on how we did it, use the comment section to contact us.